Python Modules: Importing and Using
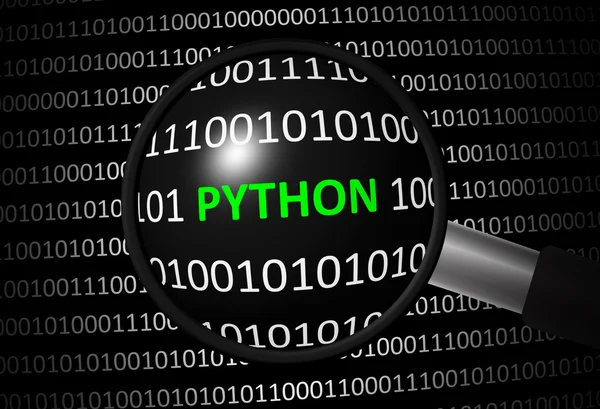
Modules in Python are files containing Python code that can be imported into other Python programs. They help in organizing and reusing code.
**Importing Modules**
You can import a module using the `import` statement:
import math
**Using Module Functions**
After importing, you can use the functions and variables defined in the module:
result = math.sqrt(16)
print(result) # Output: 4.0
**Creating Modules**
You can create your own modules by saving Python code in a `.py` file and importing it:
# my_module.py
def greet(name):
return f”Hello, {name}!”
**Importing Your Module**
You can import and use your custom module:
import my_module
print(my_module.greet(“Alice”)) # Output: Hello, Alice!
**Conclusion**
Modules are essential for organizing code and promoting code reuse. Understanding how to import and use both standard and custom modules is key for efficient Python programming.